Using Sahi to Automate testing of API’s
We previously blogged about using the testing tool Sahi to add browser testing to our CI pipeline. Today we’ll show you how we leverage the same testing tool to automate our testing of API’s that utilise the OAuth authorization protocol.
We are in a boom with the use of API’s for communication between applications. API’s provide us with a way to access data and operations without having to know anything about the underlying implementation by the developer who creates them. For those designing and developing API’s, it is ideal to automate the testing of them as it is with any application. In addition to functional testing, it is common that these API’s have security features which also need to be tested. Sometimes this can pose an impediment to creating such tests. In this article, we will discuss how we can create & perform tests on an API using the OAuth authorization protocol.
Scenario
You are developing an API which is accessed via OAuth authentication. It has a URL https://MyAPI.com/token that is accessed using a username and password. Your API will be deployed to multiple environments which take the form of subdomains. You access and test multiple copies of your application at https://[environment].MyAPI.com/token, where [environment] could be one of “dev”, “qa” or “uat” for example.
We can develop tests for this API with Sahi using data-driven suites.
Example
First, we need to get the authorization token. We create a function to do so. In this function, there is a request to the specified URL (you can see that we have configured a variable for the environment to be accessed – in the last part of the article you will see why we use variables).
Step 1
function getAuthorization(){ $request = new RESTRequest(); $request.setURL("https://" + $environment + ".MyAPI.com/token"); }
Our authentication requires a username and password, so we’ll also add those to the request. For this, we need three parameters: grant_type (which is set to the hard-coded “password” string), username and password (which are both set with variables). These three parameters are added to the body of the request.
Step 2
$p = new Parameter(); $p.add("grant_type", "password"); $request.addToBody($p); $p = new Parameter(); $p.add("username", $username); $request.addToBody($p); $p = new Parameter(); $p.add("password", $password); $request.addToBody($p);
We then send the request and receive a response:
Step 3
$response = $request.submit("post");
From our response object we get the authorization token which is returned to the function:
Step 4
$responseBody = $response.getBodyAsString(); $responseArray = JSON.parse($responseBody); $auth = "Bearer " + $responseArray["access_token"]; return $auth;
This authorization token function will be used in all API test fixtures. This token is set as the header for authorization required in all our tests. The following illustrates how we include this class and utilize the function:
Step 5
// Get the token _include("Auth.sah"); (function(){ $auth = getAuthorization();
First, we include the file where we have our authorization function, in this case, we have it saved as a file called ‘Auth.sah’. We obtain authorization by calling the function contained within – getAuthorization(). We then use the returned authorization token to consume our API:
Step 6
// Get API info $request = new RESTRequest(); $request.setURL("https://" + $environment + ".MyAPI.com/api/GetSomeInfo"); $request.setHeader("Authorization", $auth);
As you can see, we first create a request with the URL of the API operation we want to get information on. Again, the environment has been indicated by a variable. Following that, we add an authorization header whose value we have obtained from our authorisation function.
Finally, we get the request and response data which we can use for testing.
Step 7
$response = $request.submit("get"); $responseCode = $response.getresponseCode(); $responseBody = $response.getBodyAsString(); $responseArray = JSON.parse($responseBody); _assertEqual($responseArray["GetSomeInfo"], “My expected info”);
Now we can run the Sahi TestRunner on our test fixture. We do not want to launch a line of execution for each test that we want to run so we design a test suite.
In our case, it will be a data-driven test suite. Using variables allow us to use the same tests for different users and environments.
Let’s take a look at the contents of a test suite file:
Result
#file,url,username,password,environment GetSomeInfo.sah,http://localhost:9999,TestUser,t3stp4ssw0rd,dev GetOtherInfo.sah,http://localhost:9999,TestUser,t3stp4ssw0rd,dev GetEvenMoreInfo.sah,http://localhost:9999,TestUser,t3stp4ssw0rd,dev
The first line defines the fields contained in each row. The first field is the test file, the second is the URL our test will target (in this case since we are testing an API and not the UI it is irrelevant so we enter in a dummy URL). Fields 3-5 specify the variables in order: User, Password and Environment as discussed above.
In our solution, we have always used the same test user but have separated tests suites by environment. Obviously, this is customizable and it can be adapted to the needs of each application.
Happy (automated) testing!
Remember if you need assistance, have a look at out Enterprises Solutions page or use our contact form.
Alternatively, give us a call at +353 (0)1 818 2949
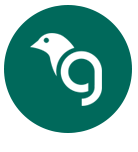
Greenfinch Technology